
JavaScript Error Free Coding: Tips to Avoid Common Mistakes
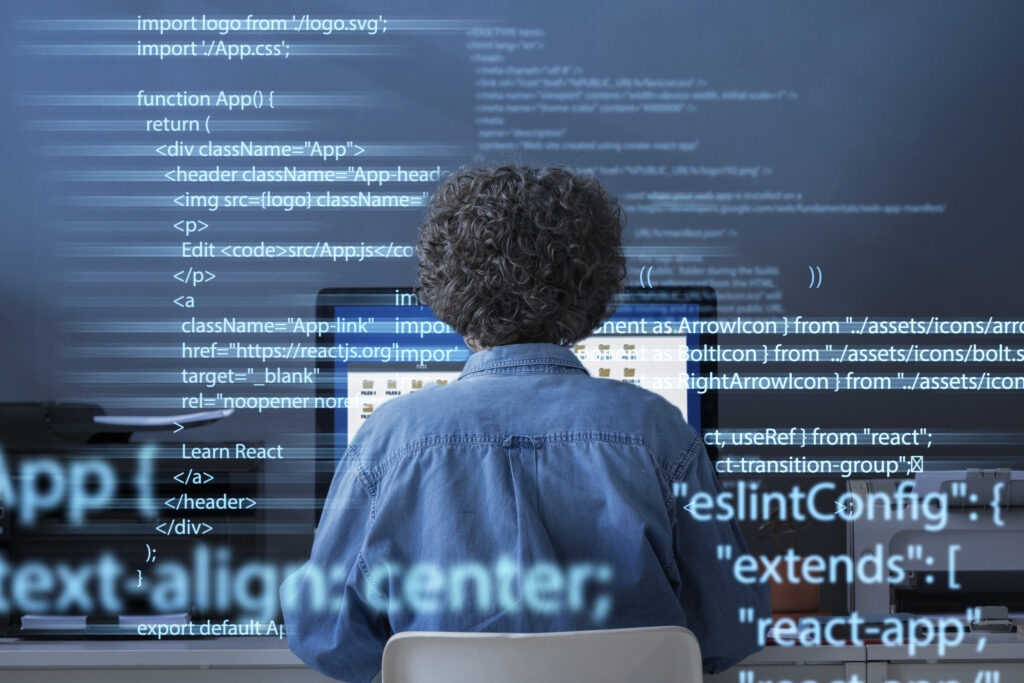
TL;DR: This article delves into common JavaScript errors that developers encounter when programming with this language. Besides listing the most frequent problems, this read focuses on each JavaScript error on this list, providing tools and tips to better understand these issues and learn how to detect and dodge them.
While JavaScript (JS) may appear simple to work with, appearances can be deceiving. From syntax-based errors to memory leaks, there are several common issues that developers may encounter when coding in this language.
Given JS’s huge popularity in modern web development, understanding these common errors and their causes is paramount for web developers. Each JavaScript error comes with an explanation that aids in identifying the problem and facilitating solutions, so don’t frown if errors have occurred or are currently happening in your code. To prevent such issues, JavaScript coding requires careful coding practices, and that’s where we are heading next.
Mastering Your JS Skills: Avoiding JavaScript Common Errors
To delve into this topic, let’s explore some of the most common errors in JavaScript that keep developers’ code from behaving as intended and look at some ways to prevent them.
Fixing Syntax-Based Errors:
Syntax errors occur when the code doesn’t conform to the expected structure and rules of the JS language. Examples typically include missing closing brackets, misspelled keywords, missing semicolons, and unterminated string literals. Let’s explore some tips to avoid syntax errors when working with JavaScript:
- Invest time in understanding the grammar of this language. Stick to clean code rules and best practices to write more readable code with fewer errors.
- Rely on tools and techniques to manage syntax-based errors. Using code editors with syntax highlighting and error checking is an excellent way to find and correct these errors while you write code.
- While indentation is not mandatory for JavaScript code to function correctly, it’s a best practice that makes the code easier to read and aids in finding and solving issues, especially in larger and more complex programs. Tools like ESLint can aid in formatting your code correctly, ensuring improved overall code quality.
Avoiding DOM-Related Errors
JavaScript errors related to the Document Object Model (DOM) occur when there are issues with manipulating or interacting with the HTML document structure.
Common DOM errors include dealing with undefined elements (manipulating or accessing non-existent DOM elements) and attempting to modify or reference attributes of elements before they’re fully loaded. To prevent unexpected behavior, avoiding these errors is crucial.
- Keep in mind that JavaScript code is executed in the order it appears in the document. Ensure that you wait for the DOM to be fully loaded before trying to access or manipulate its elements.
- You can delay script execution until the document is ready by using the DOMContentLoaded event handler. Alternatively, you can leverage JS libraries like jQuery to ensure that the DOM is fully loaded before accessing it.
- Implement error handling to prevent DOM-related errors from disrupting your application. Wrap your DOM manipulation code in try-catch blocks to gracefully handle exceptions. Remember that error handling is vital for any JavaScript application!
Dealing with Undefined or Null Keywords:
Another set of common errors in JavaScript arises from the improper usage of ‘undefined’ and ‘null’ references, often involving their interchangeable or incorrect use.
- The first step in avoiding these errors is to understand their distinctions. The ‘null’ keyword is used to assign a non-existent value to a variable or property. It serves as both an assignment value and a JavaScript object. On the flip side, ‘undefined’ indicates the absence of an assigned value to a declared variable or property, including cases where nothing has been declared at all.
- Issues can arise when trying to access variables or object properties that are either non-existent or unassigned. To address these errors, implement checks to ensure that they exist and have a value assigned to them. You can use conditional statements, such as if or typeof, to verify the presence of the variable or property before accessing it.
Ensuring Cross-Browser Compatibility
Whenever developers work on a website or web application, they may encounter cross-browser compatibility issues. These errors may arise from using modern JavaScript features unsupported by older browsers or versions or when working with unsupported native and third-party libraries in web development. To ensure cross-browser consistency:
- Check browser compatibility for JavaScript by regularly testing your website or application on a variety of real browsers, including popular ones like Chrome, Firefox, and Safari. This helps identify issues early and ensures compatibility.
- Figure out JavaScript function compatibility with older browsers and versions using tools like Can I use to identify which features are supported by specific browsers or versions. Code accordingly and use JavaScript frameworks to ease compatibility across multiple browsers.
- If you’re using advanced JavaScript features not supported in older browsers, consider transpiling your code using tools like Babel. This converts modern JS into an older version that is widely compatible.
Preventing Memory Leaks
JavaScript memory leaks happen when the application keeps references to objects that are no longer needed, preventing the JS garbage collector from freeing up memory. References to defunct objects, forgotten timers or callbacks, and circular references are some of the more common errors.
To avoid these leaks:
- Manage closures carefully: When it comes to memory leaks, closures can be a common source of them. Consider using weak references or releasing references when they’re no longer needed by setting those variables to null or undefined. This signals to the JavaScript garbage collector that they can be collected.
- You can also rely on memory profilers, such as Chrome DevTools, to identify and fix memory leaks. These tools allow you to profile your code, look for objects that are not being garbage collected, and trace their references to find the root cause.
- When attaching event listeners to DOM elements, always remember to remove them when they are no longer needed. Active event listeners can prevent all variables captured in their scope from being garbage collected, potentially leading to memory leaks.
Mitigating Issues with Strict Mode
Applying ‘strict mode’ to your JavaScript code is a great way to implement stricter parsing and error handling during runtime:
- When you enable strict mode, JavaScript engines become less forgiving of coding mistakes and raise errors in situations that would otherwise fail silently or show unexpected behavior.
- In non-strict mode, certain actions that would normally be considered mistakes are silently ignored, making it difficult to spot and fix errors. Strict mode makes these errors explicit by throwing exceptions.
- By catching potential issues early and preventing the accidental creation of global variables, the strict mode also serves to enhance the security of your code.
Related read: Regex: Simplify Your Work with JavaScript Regular Expressions
Testing as a Best Practice: A Safety Net
As JavaScript is a dynamically typed and loosely structured language, errors may not always be immediately apparent during development. Testing becomes crucial because it allows developers to systematically verify that their code works as intended and catch issues on time. So, don’t skip testing. Instead, run tests throughout the development process and address issues in advance.
Don’t Worry if You Run into These Errors from Time to Time
Always keep in mind that errors are common during coding, and even the most experienced developers are not exempt from making mistakes!
Mastering JavaScript demands careful coding practices and a deep understanding of how it works to avoid common mistakes. We hope that by following these tips and best practices, you can effectively address the problems you’ve encountered and find valuable guidance to produce clean, efficient, and reliable code.
Keep reading our blog for more useful tips and resources to develop your skills and stay ahead in your journey as a developer.