
Regex: Simplify Your Work with JavaScript Regular Expressions
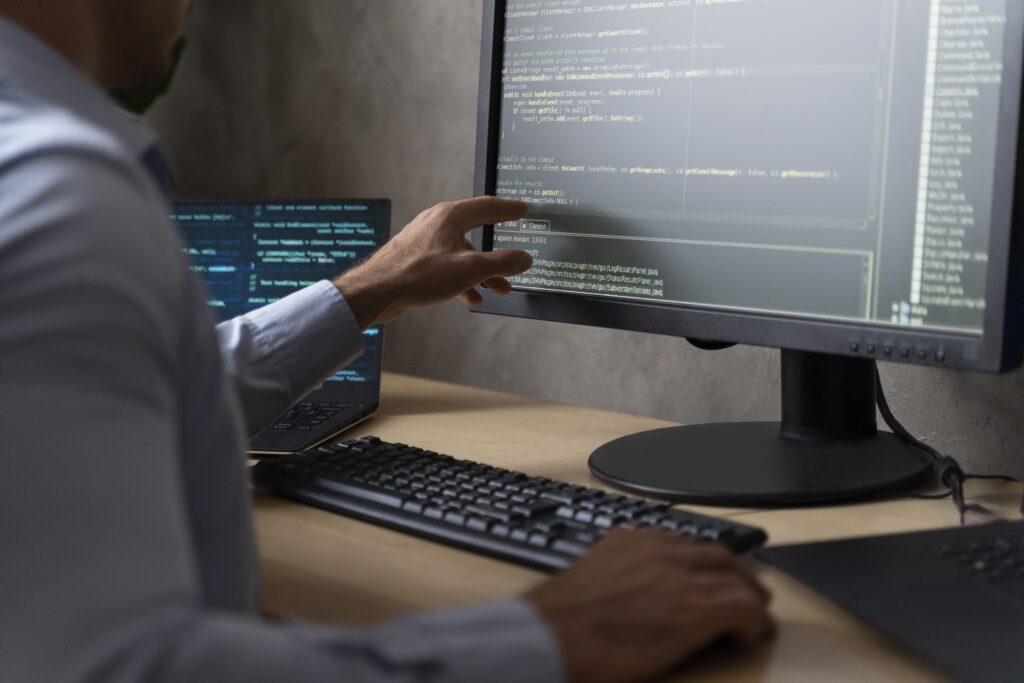
TL; DR: Regular expressions (regex) offer a robust method for managing text in JavaScript. This guide illustrates their practical applications and provides actionable examples to aid understanding. It further explores the use of online tools for testing and debugging, empowering you to effectively incorporate regex into your programming skills for more efficient and streamlined code.
In JavaScript programming, dealing with text data — known as “strings”— is an ever-present challenge. However, there’s a powerful ally that can simplify your coding and make everyday tasks easier.
Regular expressions, also known as “regex,” are a versatile tool that can inspect and manipulate strings with precision. They form a unique language within JavaScript, enabling you to describe and match patterns in string data with ease. Whether it’s handling user input, dissecting data structures like XML or JSON, or even analyzing the content of your code itself, regular expressions are a highly valuable tool.
So, what everyday JavaScript programming tasks can be simplified by using them? In this article, we’ll delve into the practical applications and examples that illustrate the potential of regular expressions in your day-to-day coding.
Exploring Regex JavaScript: What It Is and How It Works
Before we venture into the practical applications of regex, it’s crucial to grasp what exactly it is. Regex acts as a mini, highly specialized language nestled within JavaScript that facilitates dynamic and efficient text processing. Regex patterns can be employed to match, locate, and manage text in ways that standard string searches can’t compete with. The beauty of regex lies in its three core applications.
- Regex is used to conduct pattern-based searches. However, it’s not your run-of-the-mill word search. With regular expressions in JavaScript, you’re able to search for patterns that map to the expected format of the term you seek. Let’s consider a scenario where you need to hunt for all terms in a text formatted as dates (day, month, and year), but you don’t have a specific date in mind. Regex makes this task a piece of cake.
- Regular expressions are instrumental in text validation. This means that they can verify whether a certain sequence of characters aligns with the prescribed pattern. For example, you could use regex to check if user input adheres to specific criteria, such as a valid email format or a phone number in a certain style.
- Regex is effective in performing substitutions that extend beyond simple text replacement. It helps extract a portion of a pattern and use it elsewhere. This comes in handy when you want to swap out parts of a string based on certain conditions.
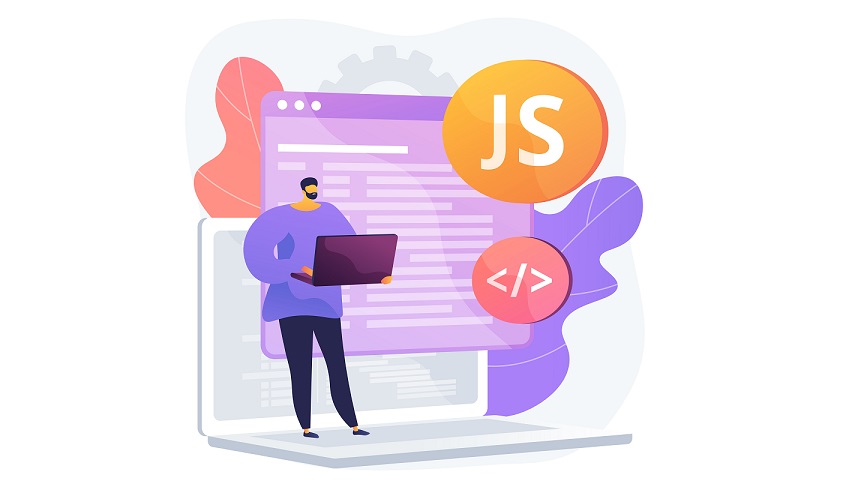
How Regex Streamlines Text Searches in Your Code
Imagine you’re working on a project that frequently involves validating user inputs, seeking or replacing matching text, or even segmenting strings into smaller sub-strings based on certain patterns. While these tasks may seem daunting, there’s a silver lining: they can all be efficiently managed with regex in JavaScript. Let’s understand how regular expressions streamline these tasks.
First up, let’s tackle a common challenge: finding all words that start with a specific letter in a text. If you were to do this manually, it would involve a significant amount of time and effort. But with regex, you can accomplish this task with ease. Consider the following example:
let wordPattern = /\ba\w*\b/g;
let testText = “Apples are amazing and avocados are awesome”;
let matches = testText.match(wordPattern); // Returns an array of words starting with ‘a’
console.log(matches);
In this case, wordPattern is the regex that matches any word starting with the letter ‘a’. We then use the match function on the testText string, which returns an array containing all the matches.
Now, consider the task of validating an email address. Traditionally, this can involve checking each character individually to ensure it adheres to the correct format — a troublesome process indeed. However, regex brings refreshing simplicity to this task. By creating a pattern that reflects the structure of a standard email address, regex can validate an input in an instant. Here’s an example:
let emailPattern = /^([a-zA-Z0-9._%-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,6})*$/;
let testEmail = “example@email.com”;
console.log(emailPattern.test(testEmail)); // Returns true if the email is valid
In this code snippet, the emailPattern variable contains the regex that describes the format of a typical email address. The test function is then used to check if the testEmail string fits this pattern.
Mastering How to Write Regex in JavaScript
Crafting regular expressions in JavaScript can feel like a form of modern art — where patterns, symbols, and a touch of creativity combine to shape a powerful tool that simplifies text processing. There are two primary methods for creating these patterns:
Method #1: Regular Expression Literals
The first method is by using a regular expression literal, which consists of a pattern enclosed within forward slashes. You can write this pattern with or without a flag (more on flags in a bit). The syntax looks like this:
const regExpLiteral = /pattern/; // Without flags
const regExpLiteralWithFlags = /pattern/g; // With flags
In this syntax, the forward slashes /…/ serve as indicators that we’re crafting a regular expression pattern. It’s analogous to how you’d use quotes “” to define a string. Flags, meanwhile, are letters added after the pattern that alter the search in some way. For instance, “g” enables a global search that finds all matches rather than stopping after the first match.
Method #2: The RegExp Constructor Function
The second method involves the use of the RegExp constructor function. Its syntax is slightly different:
new RegExp(pattern [, flags])
In this case, both the pattern and the optional flags are enclosed in quotes.
So, now that you know the two methods, how do you choose which one to use? It depends on the scenario at hand. You would typically resort to a regex literal when the regular expression pattern is known at the time of coding. This method is direct and to-the-point, making it suitable for static patterns.
On the flip side, if you need to create the regex pattern dynamically during runtime, the RegExp constructor is the way to go.
Useful Regular Expressions Online Testing Tools: RegExr and Regex101
For real-time, interactive testing and debugging, online tools like RegExr and Regex101 are excellent companions. These platforms offer a user-friendly environment to test and refine your regular expressions, providing immediate feedback and helping you understand and debug your patterns effectively.
Hands-On: Regex Examples in JavaScript
Let’s get our hands dirty and look at some practical regex examples in JavaScript, focusing on matching, grouping, replacing, splitting, and testing text.
Example 1: Matching
To match any digit in a string, you can use the \d character class in a regular expression. For instance:
const myString = ‘There are 3 apples’;
const myRegExp = /\d/;
console.log(myRegExp.test(myString)); // Output: true
This returns true if there’s any digit in the string ‘There are 3 apples’.
Example 2: Grouping
Grouping in regular expressions is accomplished using parentheses. For example, (ab)+ matches one or more occurrences of ‘ab’. Let’s look at a use case:
const myString = ‘abcabcabc’;
const myRegExp = /(abc)+/;
console.log(myRegExp.test(myString)); // Output: true
This returns true since there are multiple instances of ‘abc’ in the string ‘abcabcabc’.
Example 3: Replacing
You can leverage the replace() method in conjunction with a regular expression to replace parts of a string. For instance:
const myString = ‘Hello world’;
const myRegExp = /world/;
console.log(myString.replace(myRegExp, ‘there’)); // Output: ‘Hello there’
This will replace ‘world’ with ‘there’, returning ‘Hello there’.
Example 4: Splitting
The split(), method coupled with a regular expression, can be used to partition a string into an array of substrings. For example:
const myString = ‘Hello world’;
const myRegExp = /\s/;
console.log(myString.split(myRegExp)); // Output: [‘Hello’, ‘world’]
In this scenario, the string ‘Hello world’ is split at the space character (denoted by /\s/), resulting in the array [‘Hello’, ‘world’].
Example 5: Testing
The test() method is used to check if a particular string fits a specified regular expression pattern. For instance, to test if a string contains any uppercase letters:
const myString = ‘Hello World’;
const myRegExp = /[A-Z]/;
console.log(myRegExp.test(myString)); // Output: true
In this case, the regular expression /[A-Z]/ matches any uppercase letter. The test() method returns true as the string ‘Hello World’ does contain uppercase letters.
By understanding and applying these regular expression examples, you’ll be better equipped to handle and manipulate text within your JavaScript code, enhancing your problem-solving abilities and increasing your overall productivity.
Taking the Next Step: Deepen Your Regex Knowledge
As we’ve explored in this article, regular expressions in JavaScript are mighty tools that can drastically streamline text-related tasks. They turn complex tasks into manageable ones, contributing to cleaner, more efficient, and more readable code.
The key to mastering regex is practice and understanding when to use it efficiently and when it’s better to opt for other solutions. So, keep studying and continue delving deeper into the world of regular expressions.
But as we all know, there’s no substitute for real-world experience. That’s why we encourage you to explore the open positions at Nearsure. It’s your chance to apply your regex knowledge, among other JavaScript tools, in practical, real-world scenarios.